My first Roslyn analyzer
2 years ago I discovered how easy it is to write your own Roslyn analyzer. It was presented to me during a meeting lead by Jiří Činčura at .Net Developer Days conference.
What exactly is Roslyn Analyzer?
Roslyn Analyzer is a tool that scans your code in order to find issues with your code style, quality, design and other things. It uses .Net Compiler Platform (Roslyn) API in order to be able to analyze your code. It can check your code on syntax level as well as on semantic level. With that in mind it gives analyzer’s author a lot of capabilities in order to write versatile tools that can help programmers in their day to day work.
Meet the RandomAnalyzers.RequiredMember
With that in mind I began working on my first Roslyn analyzer. It lets you decorate members of structs/classes with `RequiredMember` attribute. By doing so you are informing the underlying analyzer that those members should be initialized every time inside an object initializer when you are creating new instance of class/struct. If you do not initialize given member then analyzer will detect that and inform you that you made a mistake and you should initialize given field:
Example Code
First let’s start by defining our class which is going to use RequiredMember attribute to mark properties/fields required during object initialization.
using RandomAnalyzers.RequiredMember;public class Person
{
public string FirstName { get; set; }
[RequiredMember]
public string LastName { get; set; }
}
When we are going to instantiate this class and provide LastName in initializer then everything is fine:
Person correctPerson = new Person
{
FirstName = "John",
LastName = "Doe"
};
Person otherCorectPerson = new Person
{
LastName = "Doe"
};
On the other hand when we omit LastName
property then compiler is going to warn us about that because analyzer signaled lack of required member initialization.
Person incorrectPerson = new Person
{
FirstName = "Federico"
};
Person otherIncorrectPerson = new Person();
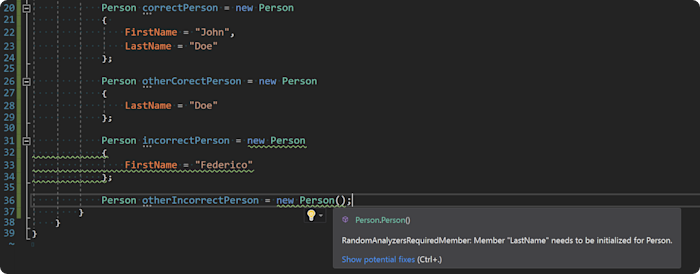
Limitations
There is one simple limitation associated with this analyzer — It cannot detect when a given property or field was initialized inside constructor. It only detects usage of given prop/field inside object initializer. One of the reasons for such behaviour is to reduce the complexity of my first analyzer.
The second reason is connected with nuget packages. Analyzer is unable to detect whether given constructor is initializing given fields inside nuget package. It is a black box for it. With that we have consistent behavior no matter whether you use nuget packages with a RequiredMember dependency or your local code.
Source Code
If you want you can check out code for my first Roslyn analyzer. It is pretty simple and it could be a good example material for anyone who wants to create their own analyzer — Click!.
Thanks for reading!
Please clap if you like the article and to learn more about services of Xfaang visit our website