Building a Real-Time Chess App with AI-Powered Commentary
Introduction
The world of chess has always been a blend of strategic depth and technological advancement. With the rise of artificial intelligence and real-time web applications, I embarked on a journey to create a simple yet engaging chess app. The idea was straightforward: develop a web-based chess game using HTML, CSS, and JavaScript that provides live AI-generated commentary on the moves as they happen.
In this blog post, I'll walk you through the process of building this application, the challenges encountered, and the solutions implemented to bring the concept to life.
The Inspiration
The inspiration behind this project was to enhance the learning and entertainment value of online chess by integrating AI-generated insights. Traditional chess apps allow players to compete against each other or AI opponents, but adding real-time commentary can elevate the user experience by:
Providing strategic insights into each move.
Offering educational content for players looking to improve.
Making the game more engaging for spectators.
Technology Stack
To keep the application accessible and lightweight, I chose to build it using:
HTML
for structuring the content.
CSS
for styling and layout.
JavaScript
for game logic and interactivity.
This stack ensures that the app runs smoothly in any modern web browser without the need for additional installations or plugins.
Building the Chessboard
HTML Structure
The chessboard is an 8x8 grid, traditionally alternating between light and dark squares. In HTML, this was achieved using a combination of <div>
elements:
<div id="chessboard">
<!-- Rows and squares will be generated dynamically -->
</div>
CSS Styling
CSS Grid was the perfect tool for creating the chessboard layout:
#chessboard {
display: grid;
grid-template-columns: repeat(8, 50px);
grid-template-rows: repeat(8, 50px);
}
.square {
width: 50px;
height: 50px;
}
.light {
background-color: #f0d9b5;
}
.dark {
background-color: #b58863;
}
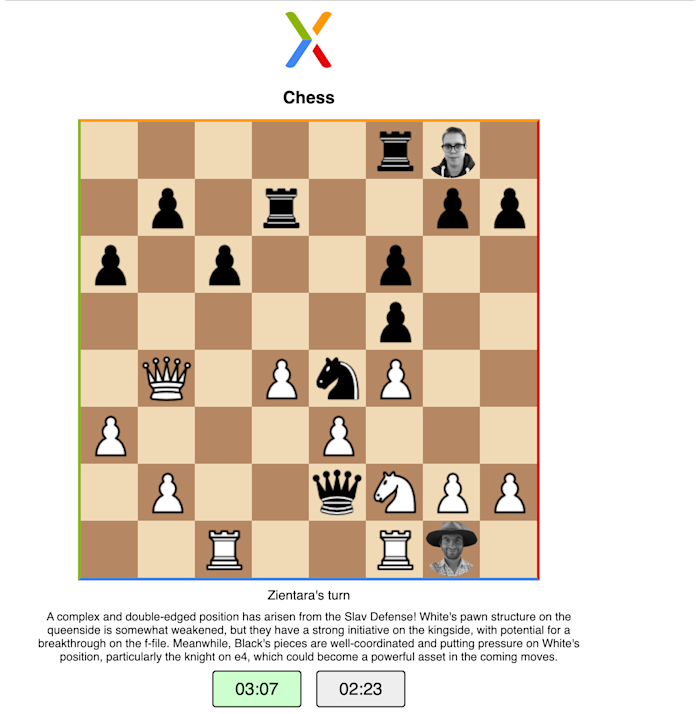
JavaScript for Dynamic Generation
Using JavaScript, the squares were generated dynamically to simplify the HTML and allow for easy adjustments:
createChessboard() {
const chessboard = document.getElementById('chessboard');
for (let row = 0; row < 8; row++) {
for (let col = 0; col < 8; col++) {
const square = document.createElement('div');
square.classList.add('square');
// Determine if the square should be light or dark
if ((row + col) % 2 === 0) {
square.classList.add('light');
} else {
square.classList.add('dark');
}
chessboard.appendChild(square);
}
}
}
createChessboard();
Implementing Game Logic
Initializing the Board State
The board state was represented as a two-dimensional array, with each element indicating the piece present on that square:
board = [
['bR', 'bN', 'bB', 'bQ', 'bK', 'bB', 'bN', 'bR'],
['bP', 'bP', 'bP', 'bP', 'bP', 'bP', 'bP', 'bP'],
['', '', '', '', '', '', '', ''],
// ... (empty rows)
['wP', 'wP', 'wP', 'wP', 'wP', 'wP', 'wP', 'wP'],
['wR', 'wN', 'wB', 'wQ', 'wK', 'wB', 'wN', 'wR']
];
Handling Moves
Players can click and drag pieces to make moves. Event listeners were added to handle user interactions:
selectedPiece = null;
function onSquareClick(row, col) {
if (selectedPiece) {
// Attempt to move the piece
movePiece(selectedPiece.row, selectedPiece.col, row, col);
selectedPiece = null;
} else if (board[row][col]) {
// Select a piece to move
selectedPiece = { row, col };
}
}
Validating Moves
To ensure the game follows chess rules, move validation was essential. A function was implemented to check the legality of each move:
isValidMove(fromRow, fromCol, toRow, toCol) {
// Implement specific rules for each piece type
const piece = board[fromRow][fromCol];
// ... (validation logic)
return true; // or false
}
Integrating AI Commentary
Setting Up the AI API
To generate AI-powered comments, an API endpoint was set up to receive the game's move history and return insightful commentary.
sendMoveHistoryToAPI(moveHistory) {
fetch('https://api.example.com/chess/commentary', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ moves: moveHistory })
})
.then(response => response.json())
.then(data => {
displayAICommentary(data.commentary);
})
.catch(error => {
console.error('Error fetching AI commentary:', error);
});
}
Triggering Commentary Updates
After each move, the move history is sent to the API:
javascriptCopy codefunction movePiece(fromRow, fromCol, toRow, toCol) { // ... (move logic) moveHistory.push({ fromRow, fromCol, toRow, toCol, piece }); sendMoveHistoryToAPI(moveHistory); }
Challenges Faced
Move Validation Complexity
Implementing complete chess move validation is complex due to the intricate rules:
Initial Approach:
Started with basic move rules for each piece.
Enhancements:
Gradually added special moves like castling, en passant, and pawn promotion.
AI Integration
Ensuring timely and relevant AI commentary required:
Optimizing API Calls:
Batched move history to minimize the number of requests.
Handling Latency:
Implemented asynchronous functions to prevent the UI from freezing while waiting for API responses.
Enhancing the User Experience
Responsive Design
Used CSS media queries to ensure the app is accessible on various screen sizes, from desktops to mobile devices.
Visual Feedback
Added highlights to indicate selected pieces and valid move options to guide the player.
Interactive Move List
Displayed the move history on the interface, allowing players to review and analyze past moves.
Conclusion and Future Work
Creating this chess app was an enriching experience that combined web development with AI capabilities. The project's simplicity in terms of technology stack did not limit its potential for providing an engaging user experience.
Future Enhancements
Improved AI Analysis:
Incorporate deeper analysis, such as evaluating board positions and suggesting potential strategies.
Multiplayer Support:
Enable online play against other users with real-time updates.
Undo and Replay Features:
Allow players to undo moves and replay games for learning purposes.
Final Thoughts
This project demonstrates how combining fundamental web technologies with AI can create interactive and educational applications. By keeping the core development simple with HTML, CSS, and JavaScript, it's accessible for developers looking to explore similar integrations without needing extensive resources.
Whether you're a chess enthusiast or a developer interested in AI applications, this project showcases the possibilities that emerge when technology and creativity intersect.
Thank you for reading! If you have any questions or suggestions, feel free to reach out! hello@xfaang.com